Simple problems, simple solutions, great joy
I’ve been coding for many, many years. I started when I was 13, and that is decades ago. Software development was different back then; programs ran on a single PC, claiming it completely, with text based interfaces (windows were drawn with characters), and maybe, just maybe, there was a shared storage, because some PC had it’s drive shared.
It was also the time when there were not many existing software systems. You had WordPerfect (no WYSIWYG), I remember some kind of simple spreadsheet, and basic bookkeeping software. But that was about it. The world, and all the potential software had, was wide open.
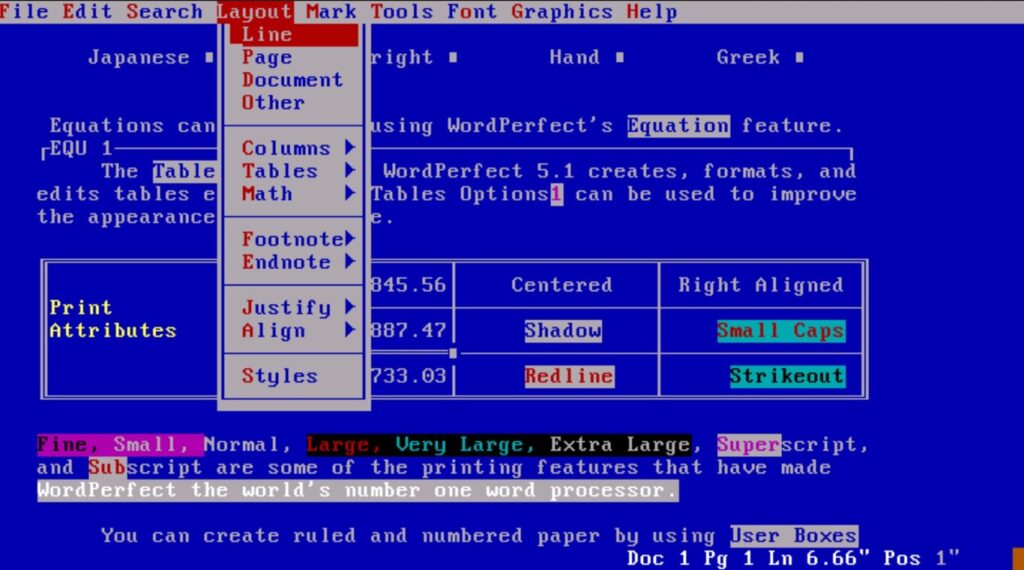
So in the years that followed I wrote many systems. Small ones, to solve small but real problems; an address register for myself, a CRM tool for the local gym, a similar one for the dance school. What a time! It was not much work, especially after MSAcesss was introduced, you got a lot of functionality for the amount of effort you had put in.
(more…)